笔记
单击此处 下载完整的示例代码
pcolormesh 网格和阴影#
axes.Axes.pcolormesh
并pcolor
有一些关于如何布置网格和网格点之间的阴影的选项。
通常,如果Z具有形状(M, N)则网格X和Y可以使用形状(M+1, N+1)或(M, N)指定,具体取决于shading
关键字参数的参数。请注意,下面我们将向量x指定为长度 N 或 N+1,将y指定为长度 M 或 M+1,并
在内部根据输入向量pcolormesh
生成网格矩阵X和Y。
import matplotlib.pyplot as plt
import numpy as np
平面着色#
具有最少假设的网格规范是shading='flat'
,如果网格比每个维度中的数据大一,即具有形状
(M+1, N+1)。在这种情况下,X和Y指定用Z中的值着色的四边形的角。在这里,我们
用X和Y指定(3, 5)四边形的边,即(4, 6)。
nrows = 3
ncols = 5
Z = np.arange(nrows * ncols).reshape(nrows, ncols)
x = np.arange(ncols + 1)
y = np.arange(nrows + 1)
fig, ax = plt.subplots()
ax.pcolormesh(x, y, Z, shading='flat', vmin=Z.min(), vmax=Z.max())
def _annotate(ax, x, y, title):
# this all gets repeated below:
X, Y = np.meshgrid(x, y)
ax.plot(X.flat, Y.flat, 'o', color='m')
ax.set_xlim(-0.7, 5.2)
ax.set_ylim(-0.7, 3.2)
ax.set_title(title)
_annotate(ax, x, y, "shading='flat'")
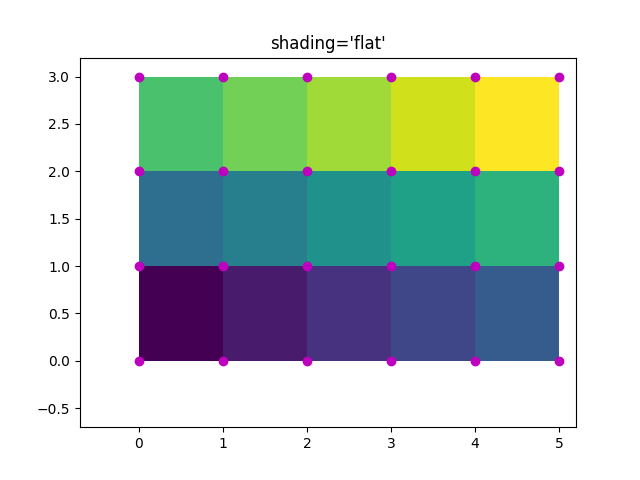
平面着色,相同形状的网格#
但是,通常会在X和Y与Z的形状匹配的情况下提供数据。虽然这对其他类型有意义shading
,但不再允许何时shading='flat'
(并且从 Matplotlib v3.3 开始会引发 MatplotlibDeprecationWarning)。从历史上看,Matplotlib 在这种情况下默默地删除了Z的最后一行和最后一列,以匹配 Matlab 的行为。如果仍需要此行为,只需手动删除最后一行和最后一列:
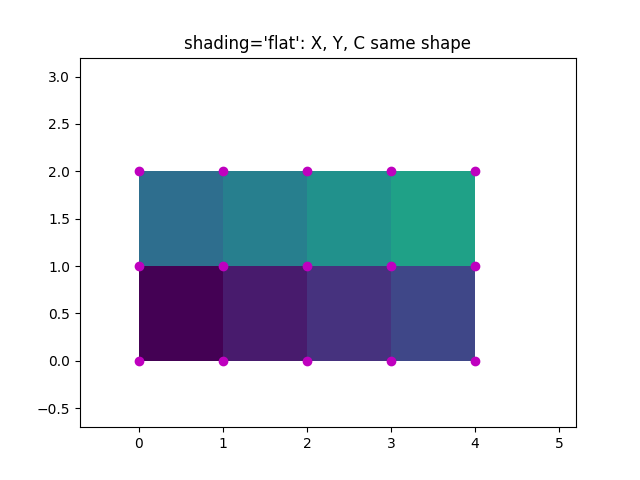
最近的阴影,相同形状的网格#
通常,当用户使X、Y和Z形状相同时,删除一行和一列数据并不是用户的意思。对于这种情况,Matplotlib 允许shading='nearest'
将彩色四边形置于网格点的中心。
如果传递的网格不是正确的形状,shading='nearest'
则会引发错误。
fig, ax = plt.subplots()
ax.pcolormesh(x, y, Z, shading='nearest', vmin=Z.min(), vmax=Z.max())
_annotate(ax, x, y, "shading='nearest'")
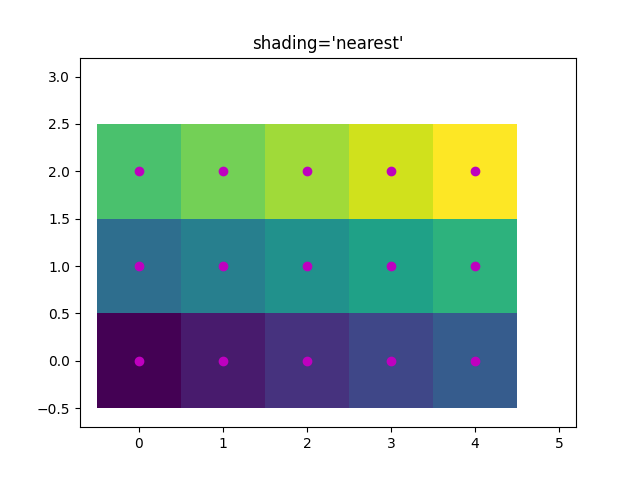
自动着色#
用户可能希望代码自动选择使用哪个,在这种情况下,将根据X、Y和Zshading='auto'
的形状决定是使用“平面”还是“最近”着色。
fig, axs = plt.subplots(2, 1, constrained_layout=True)
ax = axs[0]
x = np.arange(ncols)
y = np.arange(nrows)
ax.pcolormesh(x, y, Z, shading='auto', vmin=Z.min(), vmax=Z.max())
_annotate(ax, x, y, "shading='auto'; X, Y, Z: same shape (nearest)")
ax = axs[1]
x = np.arange(ncols + 1)
y = np.arange(nrows + 1)
ax.pcolormesh(x, y, Z, shading='auto', vmin=Z.min(), vmax=Z.max())
_annotate(ax, x, y, "shading='auto'; X, Y one larger than Z (flat)")
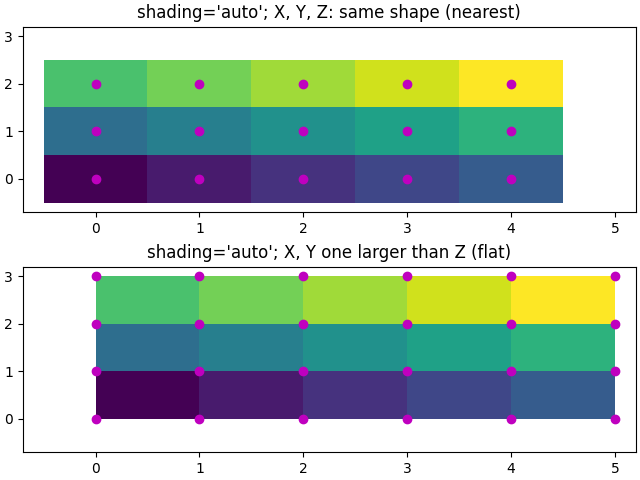
Gouraud 着色#
也可以指定Gouraud 着色,其中四边形中的颜色在网格点之间线性插值。X , Y , Z的形状必须相同。
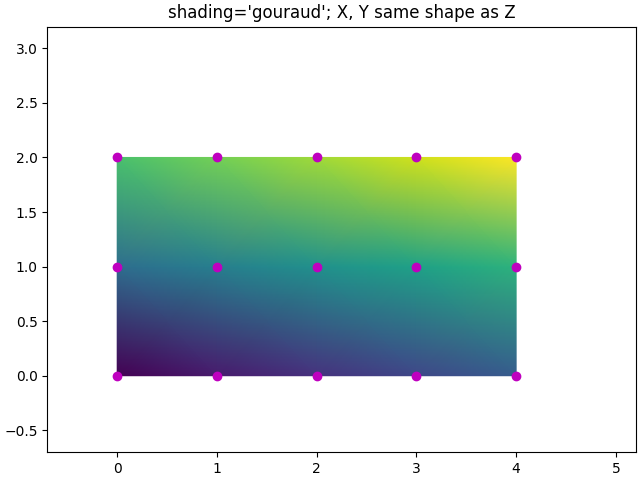
脚本总运行时间:(0分2.324秒)