笔记
单击此处 下载完整的示例代码
暂停和恢复动画#
这个例子展示了:
使用 Animation.pause() 方法暂停动画。
使用 Animation.resume() 方法恢复动画。
笔记
这个例子练习了 Matplotlib 的交互能力,这不会出现在静态文档中。请在您的机器上运行此代码以查看交互性。
您可以复制和粘贴单个部分,或使用页面底部的链接下载整个示例。
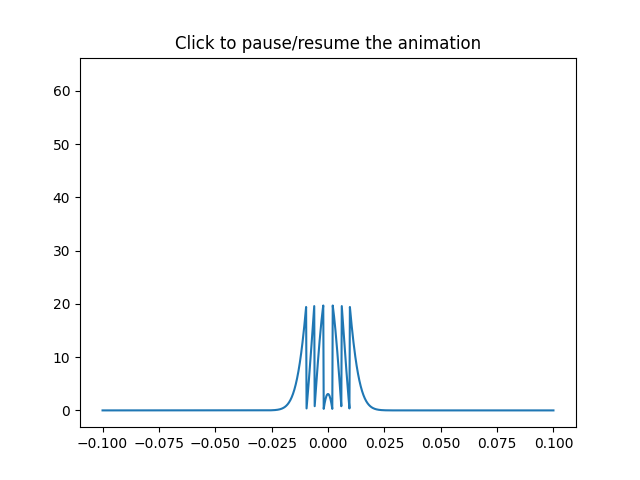
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
class PauseAnimation:
def __init__(self):
fig, ax = plt.subplots()
ax.set_title('Click to pause/resume the animation')
x = np.linspace(-0.1, 0.1, 1000)
# Start with a normal distribution
self.n0 = (1.0 / ((4 * np.pi * 2e-4 * 0.1) ** 0.5)
* np.exp(-x ** 2 / (4 * 2e-4 * 0.1)))
self.p, = ax.plot(x, self.n0)
self.animation = animation.FuncAnimation(
fig, self.update, frames=200, interval=50, blit=True)
self.paused = False
fig.canvas.mpl_connect('button_press_event', self.toggle_pause)
def toggle_pause(self, *args, **kwargs):
if self.paused:
self.animation.resume()
else:
self.animation.pause()
self.paused = not self.paused
def update(self, i):
self.n0 += i / 100 % 5
self.p.set_ydata(self.n0 % 20)
return (self.p,)
pa = PauseAnimation()
plt.show()