笔记
单击此处 下载完整的示例代码
自定义框样式#
此示例演示自定义的实现BoxStyle
。Custom ConnectionStyle
s 和ArrowStyle
s 可以类似地定义。
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
自定义框样式可以实现为一个函数,该函数接受指定矩形框和“变异”数量的参数,并返回“变异”路径。具体签名是以下之一
custom_box_style
。
在这里,我们返回一个新路径,它在框的左侧添加了一个“箭头”形状。
然后可以通过传递
来使用自定义框样式。bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
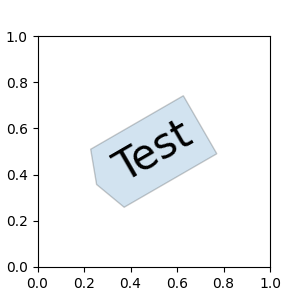
Text(0.5, 0.5, 'Test')
同样,自定义框样式可以实现为实现
__call__
.
然后可以将这些类注册到BoxStyle._style_list
dict 中,这允许将框样式指定为字符串,
. 请注意,此注册依赖于内部 API,因此不受官方支持。bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
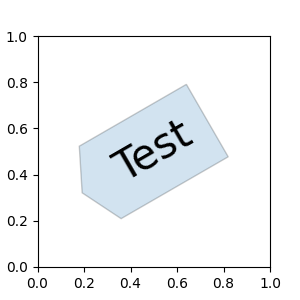